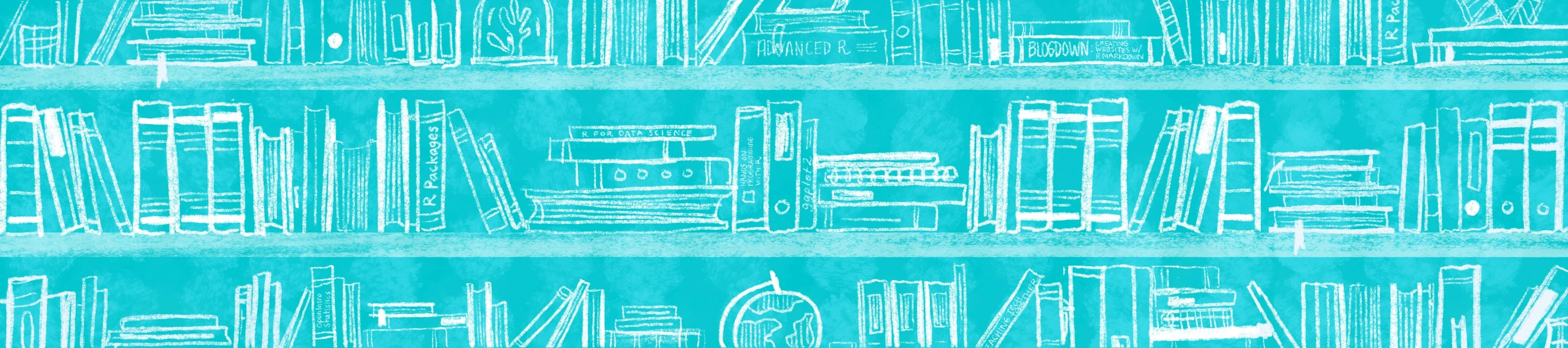
פרק 2 - לולאות
לולאה היא מבנה שמאפשר להריץ קטע קוד מספר פעמים, בצורה חזרתית.
לולאת for
איטרציה (iteration) היא פעולה שמתארת מעבר על אובייקט שמכיל מספר איברים, איבר אחרי איבר. בהתחלה האיבר הראשון, השני, השלישי וכן הלאה. לולאת for מבצעת איטרציה על אובייקט (בד”כ וקטור) כאשר בכל מחזור ריצה של הלולאה חוזר ערך אחר.
מבנה כללי:
for (value in sequence) {
*loop body* #the code here will run every time the loop iterate
}
בדוגמא הבאה ניתן לראות לולאה שעושה איטרציה על וקטור של מספרים מ1 עד 7 ולכן רצה בדיוק שבע פעמים: שימי לב שבכל מחזור ריצה של הלולאה המשתנה i מקבל את הערך הנוכחי שחזר מהוקטור.
for (i in 1:7) {
print(i)
}
## [1] 1
## [1] 2
## [1] 3
## [1] 4
## [1] 5
## [1] 6
## [1] 7
עוד דוגמא: לולאת for שעושה איטרציה על וקטור של משתנה מסוג “character”
<- c('lion','monkey','tiger')
animals for (a in animals) {
print(a)
}
## [1] "lion"
## [1] "monkey"
## [1] "tiger"
דוגמא 3:
# using i as index
<- c('lion','monkey','tiger')
animals <- c('apple','orange','pineapple')
fruits for (i in 1:length(animals)) {
print(paste(i, animals[i], fruits[i]))
}
## [1] "1 lion apple"
## [1] "2 monkey orange"
## [1] "3 tiger pineapple"
דוגמא 4: יצירת לוח הכפל בעזרת שתי לולאות for אחת בתוך השנייה
# creates a 10X10 matrix contains only NA values
<- matrix(nrow = 10, ncol = 10)
mul_table <- nrow(mul_table) # 10 rows
row_num <- ncol(mul_table) # 10 columns
col_num
for (i in 1:row_num) {
for (j in 1:col_num) {
#print(paste('i:',i,'j:',j))
<- i*j # each square will contain the multiplication of the row number with the col number
mul_table[i,j]
}
}print(mul_table)
## [,1] [,2] [,3] [,4] [,5] [,6] [,7] [,8] [,9] [,10]
## [1,] 1 2 3 4 5 6 7 8 9 10
## [2,] 2 4 6 8 10 12 14 16 18 20
## [3,] 3 6 9 12 15 18 21 24 27 30
## [4,] 4 8 12 16 20 24 28 32 36 40
## [5,] 5 10 15 20 25 30 35 40 45 50
## [6,] 6 12 18 24 30 36 42 48 54 60
## [7,] 7 14 21 28 35 42 49 56 63 70
## [8,] 8 16 24 32 40 48 56 64 72 80
## [9,] 9 18 27 36 45 54 63 72 81 90
## [10,] 10 20 30 40 50 60 70 80 90 100
לולאת while
לולאה שרצה כל עוד תנאי מסוים מתקיים.
מבנה כללי:
while (condition) {
*loop body* #the code here will run every time the loop iterate,
#while the condition returns TRUE
}
דוגמא 1: הלולאה הבאה רצה כל עוד המשתנה i קטן מ5. בכל ריצה של הלולאה i יגדל ב-1 כך שהלולאה תרוץ בדיוק 4 פעמים.
<- 1
i while (i < 5) {
print (i)
<- i + 1
i }
## [1] 1
## [1] 2
## [1] 3
## [1] 4
דוגמא 2: לולאת while עם שלושה תנאים:
<- 1
index while (index < 7) {
# if the remain of dividing "index" by 2 is 0
if (index %% 2 == 0) {
# do this:
print(paste('the number',index, 'is even'))
}# else, if "index" is 5
else if (index == 5) {
# do this:
print('5!')
}# else, if the remain of dividing "index" by 2 is not 0
else if (index %% 2 != 0) {
# do this:
print(paste('the number',index, 'is not even and not 5'))
}<- index + 1
index }
## [1] "the number 1 is not even and not 5"
## [1] "the number 2 is even"
## [1] "the number 3 is not even and not 5"
## [1] "the number 4 is even"
## [1] "5!"
## [1] "the number 6 is even"
לולאת repeat
זאת הלולאה הפשוטה ביותר - קטע קוד שרץ פעם אחר פעם. חשוב שיהיה ללולאת repeat תנאי עצירה, אחרת היא תמשיך לרוץ לנצח…
מבנה כללי:
repeat {
*loop body* #the code here will run time after time
if (*stopping condition*) {
break
}
}
דוגמא: לולאה שמדפיסה את כל המספרים הזוגיים עד 10.
= 0
i repeat {
<- i + 2
i print(i)
if (i == 10) {
break
} }
## [1] 2
## [1] 4
## [1] 6
## [1] 8
## [1] 10